因为自己擅长的是php,所以用之前发的文章python采集到内容之后使用http接口方便php调用和处理。
pip install tornado
import tornado.ioloop
import tornado.web
class MainHandler(tornado.web.RequestHandler):
def get(self):
"""get请求"""
a = self.get_argument('a')
b = self.get_argument('b')
c = int(a) + int(b)
self.write("c=" + str(c))
application = tornado.web.Application([(r"/add", MainHandler), ])
if __name__ == "__main__":
application.listen(8868)
tornado.ioloop.IOLoop.instance().start()
接口访问:http://127.0.0.1:8868/add?a=1&b=2
python文件调用其他.py文件的函数
如果A.py文件与B.py文件在同一个文件夹下:(A.py调用B.py的函数或者类)
B.py的函数:
def add(x,y):
z=x+y
return z
A.py文件调用函数
from B import add
sum=add(4,5)
##或者
import B
sum=B.add(4,5)
B.py的类
class sum():
def init(self,x,y):
self.x=x
self.y=y
def add(self):
sum=self.x+self.y
return sum
A.py文件调用类
from B import sum
get_sum=sum(4,5)
value=get_sum.add()
##或者
import B
get_sum=B.sum(4,5)
value=get_sum.add()
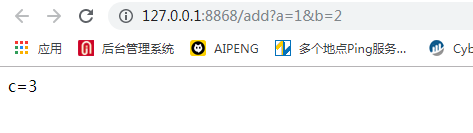